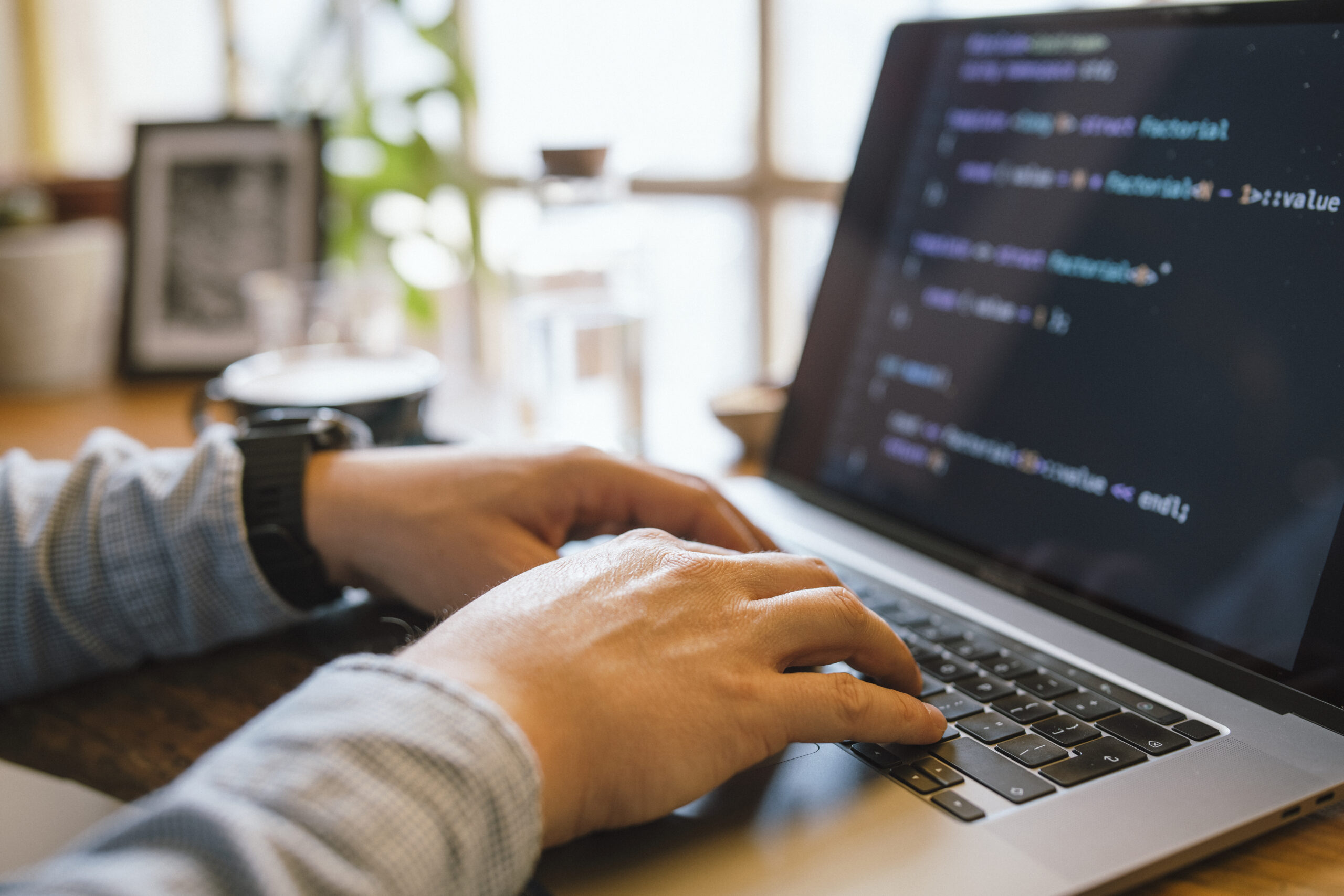
Debugging is Probably the most necessary — yet usually neglected — competencies inside a developer’s toolkit. It is not just about fixing broken code; it’s about comprehension how and why points go wrong, and Understanding to Believe methodically to solve difficulties successfully. Regardless of whether you're a novice or perhaps a seasoned developer, sharpening your debugging competencies can help save hours of annoyance and dramatically boost your productivity. Listed here are numerous approaches to help developers amount up their debugging recreation by me, Gustavo Woltmann.
Grasp Your Instruments
On the list of fastest methods builders can elevate their debugging abilities is by mastering the equipment they use everyday. While composing code is just one part of advancement, realizing the best way to communicate with it correctly all through execution is Similarly important. Modern day advancement environments arrive equipped with potent debugging capabilities — but several developers only scratch the area of what these equipment can do.
Choose, one example is, an Built-in Growth Atmosphere (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These applications enable you to set breakpoints, inspect the value of variables at runtime, stage through code line by line, and in many cases modify code about the fly. When used the right way, they Enable you to observe precisely how your code behaves all through execution, that's invaluable for tracking down elusive bugs.
Browser developer applications, such as Chrome DevTools, are indispensable for entrance-end builders. They permit you to inspect the DOM, monitor network requests, see actual-time overall performance metrics, and debug JavaScript in the browser. Mastering the console, resources, and community tabs can change frustrating UI issues into manageable responsibilities.
For backend or technique-level builders, resources like GDB (GNU Debugger), Valgrind, or LLDB offer deep Regulate more than jogging processes and memory management. Discovering these tools might have a steeper Mastering curve but pays off when debugging overall performance troubles, memory leaks, or segmentation faults.
Further than your IDE or debugger, turn out to be relaxed with Variation Management units like Git to know code historical past, obtain the precise moment bugs were being introduced, and isolate problematic modifications.
Eventually, mastering your instruments usually means heading beyond default options and shortcuts — it’s about producing an personal expertise in your enhancement environment in order that when issues arise, you’re not misplaced at midnight. The better you already know your applications, the greater time you are able to invest solving the actual difficulty as opposed to fumbling by means of the process.
Reproduce the Problem
Just about the most essential — and infrequently forgotten — steps in successful debugging is reproducing the trouble. Before jumping in to the code or generating guesses, builders have to have to produce a steady setting or situation the place the bug reliably appears. Without having reproducibility, fixing a bug becomes a recreation of opportunity, often bringing about squandered time and fragile code adjustments.
The first step in reproducing a dilemma is gathering just as much context as is possible. Request questions like: What steps resulted in The difficulty? Which ecosystem was it in — progress, staging, or generation? Are there any logs, screenshots, or error messages? The greater depth you've got, the simpler it becomes to isolate the precise disorders underneath which the bug happens.
Once you’ve gathered sufficient information, attempt to recreate the problem in your neighborhood atmosphere. This may suggest inputting the identical information, simulating equivalent user interactions, or mimicking process states. If the issue seems intermittently, contemplate writing automated tests that replicate the sting instances or condition transitions associated. These exams not simply assist expose the problem and also stop regressions Sooner or later.
In some cases, the issue can be environment-unique — it would transpire only on certain working devices, browsers, or below unique configurations. Utilizing applications like virtual machines, containerization (e.g., Docker), or cross-browser screening platforms is often instrumental in replicating such bugs.
Reproducing the trouble isn’t merely a stage — it’s a frame of mind. It requires persistence, observation, and also a methodical approach. But when you can constantly recreate the bug, you happen to be currently halfway to fixing it. By using a reproducible circumstance, You may use your debugging applications more properly, take a look at probable fixes properly, and connect additional Evidently with all your team or end users. It turns an summary grievance right into a concrete problem — and that’s in which developers thrive.
Examine and Have an understanding of the Mistake Messages
Mistake messages are sometimes the most useful clues a developer has when anything goes Mistaken. In lieu of observing them as annoying interruptions, developers ought to learn to take care of mistake messages as direct communications through the program. They frequently tell you what precisely transpired, the place it occurred, and at times even why it took place — if you know how to interpret them.
Start by looking at the concept cautiously As well as in entire. Numerous developers, especially when underneath time stress, look at the primary line and right away begin making assumptions. But further while in the error stack or logs may well lie the real root trigger. Don’t just duplicate and paste error messages into search engines like google — go through and understand them 1st.
Break the error down into parts. Could it be a syntax mistake, a runtime exception, or maybe a logic error? Will it position to a specific file and line range? What module or operate triggered it? These issues can guidebook your investigation and issue you towards the responsible code.
It’s also valuable to understand the terminology on the programming language or framework you’re using. Error messages in languages like Python, JavaScript, or Java normally adhere to predictable patterns, and Mastering to recognize these can substantially speed up your debugging method.
Some glitches are vague or generic, and in those circumstances, it’s very important to examine the context during which the mistake happened. Check out similar log entries, input values, and up to date changes inside the codebase.
Don’t forget compiler or linter warnings possibly. These frequently precede more substantial difficulties and supply hints about probable bugs.
Finally, error messages usually are not your enemies—they’re your guides. Finding out to interpret them effectively turns chaos into clarity, helping you pinpoint problems more quickly, lessen debugging time, and become a more effective and self-confident developer.
Use Logging Correctly
Logging is Among the most impressive tools inside a developer’s debugging toolkit. When utilised proficiently, it offers authentic-time insights into how an software behaves, serving to you fully grasp what’s going on underneath the hood without having to pause execution or stage with the code line by line.
A great logging technique starts with understanding what to log and at what level. Common logging levels involve DEBUG, Information, WARN, Mistake, and Lethal. Use DEBUG for specific diagnostic facts all through progress, Details for standard situations (like thriving start out-ups), WARN for prospective problems that don’t crack the applying, ERROR for real difficulties, and FATAL in the event the technique can’t proceed.
Steer clear of flooding your logs with excessive or irrelevant details. Far too much logging can obscure significant messages and decelerate your program. Focus on vital functions, state variations, input/output values, and critical final decision details within your code.
Structure your log messages Plainly and regularly. Involve context, for example timestamps, request IDs, and performance names, so it’s easier to trace challenges in dispersed systems or multi-threaded environments. Structured logging (e.g., JSON logs) may make it even easier to parse and filter logs programmatically.
Through debugging, logs Allow you to keep track of how variables here evolve, what situations are achieved, and what branches of logic are executed—all without having halting the program. They’re Primarily useful in output environments in which stepping as a result of code isn’t achievable.
In addition, use logging frameworks and instruments (like Log4j, Winston, or Python’s logging module) that help log rotation, filtering, and integration with monitoring dashboards.
In the long run, wise logging is about equilibrium and clarity. Using a perfectly-believed-out logging tactic, you are able to decrease the time it's going to take to identify challenges, obtain further visibility into your applications, and Enhance the Over-all maintainability and reliability of the code.
Imagine Just like a Detective
Debugging is not merely a technical process—it is a form of investigation. To proficiently identify and resolve bugs, builders ought to strategy the procedure similar to a detective solving a mystery. This attitude can help stop working complicated troubles into workable components and stick to clues logically to uncover the basis bring about.
Get started by accumulating proof. Look at the signs and symptoms of the challenge: mistake messages, incorrect output, or overall performance concerns. Similar to a detective surveys a criminal offense scene, accumulate just as much suitable facts as you could without jumping to conclusions. Use logs, examination situations, and consumer studies to piece collectively a clear picture of what’s happening.
Next, form hypotheses. Ask by yourself: What may be resulting in this habits? Have any alterations just lately been manufactured to your codebase? Has this situation transpired prior to under comparable situations? The objective is to slender down options and discover prospective culprits.
Then, check your theories systematically. Try to recreate the condition in a managed surroundings. In the event you suspect a selected operate or component, isolate it and validate if The problem persists. Like a detective conducting interviews, check with your code queries and Allow the results direct you closer to the reality.
Pay out shut consideration to little aspects. Bugs typically conceal in the minimum expected destinations—like a lacking semicolon, an off-by-one particular error, or maybe a race problem. Be complete and affected individual, resisting the urge to patch the issue without the need of completely understanding it. Short term fixes may conceal the actual issue, just for it to resurface later.
And finally, preserve notes on Anything you attempted and figured out. Just as detectives log their investigations, documenting your debugging process can preserve time for future troubles and assistance Other individuals fully grasp your reasoning.
By contemplating similar to a detective, developers can sharpen their analytical expertise, tactic problems methodically, and grow to be more practical at uncovering concealed problems in intricate devices.
Compose Assessments
Crafting tests is one of the best solutions to improve your debugging expertise and Total enhancement efficiency. Tests not simply enable capture bugs early but also serve as a safety net that gives you self-assurance when generating improvements on your codebase. A perfectly-tested application is easier to debug since it permits you to pinpoint just the place and when a challenge happens.
Begin with unit exams, which give attention to unique capabilities or modules. These compact, isolated checks can promptly expose irrespective of whether a selected bit of logic is Doing work as anticipated. Each time a check fails, you quickly know the place to seem, substantially lowering the time spent debugging. Device checks are Primarily handy for catching regression bugs—troubles that reappear right after Earlier getting set.
Next, combine integration exams and finish-to-end checks into your workflow. These support make certain that numerous aspects of your software get the job done collectively smoothly. They’re significantly valuable for catching bugs that happen in complex devices with several factors or expert services interacting. If one thing breaks, your checks can show you which Section of the pipeline failed and underneath what circumstances.
Crafting exams also forces you to definitely Consider critically about your code. To test a feature adequately, you'll need to know its inputs, predicted outputs, and edge instances. This volume of knowing The natural way qualified prospects to raised code construction and much less bugs.
When debugging an issue, composing a failing test that reproduces the bug could be a robust initial step. As soon as the check fails continually, you are able to center on correcting the bug and observe your take a look at go when the issue is solved. This solution ensures that a similar bug doesn’t return in the future.
In a nutshell, crafting tests turns debugging from a aggravating guessing activity right into a structured and predictable procedure—supporting you capture extra bugs, quicker and a lot more reliably.
Take Breaks
When debugging a tricky concern, it’s uncomplicated to be immersed in the problem—looking at your display for hrs, striving Option just after solution. But Probably the most underrated debugging resources is just stepping absent. Getting breaks can help you reset your intellect, cut down frustration, and often see the issue from a new perspective.
If you're much too near the code for much too long, cognitive fatigue sets in. You could commence overlooking apparent mistakes or misreading code which you wrote just hours earlier. In this point out, your Mind will become a lot less successful at dilemma-fixing. A short walk, a coffee crack, or maybe switching to a distinct activity for 10–quarter-hour can refresh your concentration. A lot of developers report discovering the root of a dilemma once they've taken time and energy to disconnect, allowing their subconscious function in the history.
Breaks also support avoid burnout, Particularly during extended debugging periods. Sitting before a display, mentally trapped, is not merely unproductive but additionally draining. Stepping away allows you to return with renewed Electricity plus a clearer state of mind. You might quickly observe a missing semicolon, a logic flaw, or simply a misplaced variable that eluded you ahead of.
In the event you’re trapped, a great general guideline is always to established a timer—debug actively for forty five–60 minutes, then have a five–10 moment break. Use that point to move all around, stretch, or do anything unrelated to code. It may come to feel counterintuitive, especially beneath limited deadlines, nonetheless it really brings about quicker and more practical debugging in the long run.
In a nutshell, having breaks just isn't an indication of weakness—it’s a wise tactic. It gives your brain Place to breathe, increases your viewpoint, and allows you avoid the tunnel eyesight That always blocks your progress. Debugging is actually a psychological puzzle, and relaxation is part of fixing it.
Study From Each Bug
Each and every bug you face is a lot more than just a temporary setback—It truly is a possibility to expand for a developer. Whether it’s a syntax error, a logic flaw, or even a deep architectural challenge, every one can teach you one thing precious for those who make an effort to reflect and assess what went wrong.
Begin by inquiring you a number of critical thoughts once the bug is resolved: What triggered it? Why did it go unnoticed? Could it are already caught previously with superior tactics like device tests, code assessments, or logging? The responses often expose blind places in the workflow or understanding and allow you to Create more robust coding behaviors transferring forward.
Documenting bugs can be a superb behavior. Preserve a developer journal or preserve a log where you note down bugs you’ve encountered, the way you solved them, and That which you figured out. After a while, you’ll start to see patterns—recurring issues or typical errors—that you can proactively stay clear of.
In staff environments, sharing Whatever you've realized from a bug with all your friends could be Particularly powerful. Irrespective of whether it’s by way of a Slack message, a brief publish-up, or a quick awareness-sharing session, serving to Some others stay away from the same challenge boosts crew efficiency and cultivates a more robust Understanding culture.
Additional importantly, viewing bugs as lessons shifts your mindset from annoyance to curiosity. As opposed to dreading bugs, you’ll start appreciating them as necessary elements of your enhancement journey. All things considered, a few of the most effective developers usually are not those who compose fantastic code, but people who consistently find out from their issues.
Ultimately, Each individual bug you resolve provides a brand new layer on your skill set. So upcoming time you squash a bug, take a second to replicate—you’ll occur away a smarter, additional capable developer as a consequence of it.
Summary
Bettering your debugging competencies requires time, exercise, and tolerance — nevertheless the payoff is big. It will make you a more productive, self-assured, and able developer. The next time you happen to be knee-deep inside of a mysterious bug, keep in mind: debugging isn’t a chore — it’s a chance to be better at Everything you do.